Script for importing with Geometry Nodes in Blender 4.2.
It did not work in my blender 4.2 environment despite trying various add-ons, but Vaticinator’s YouTube helped me solve the problem!
My script creates the Geo-Nodes configuration introduced by Vaticinator in one shot.
YouTube by Vaticinator for reference.
https://www.youtube.com/watch?v=KRxDyloJRYU
もくじ
Obj files loading process
Create a new collection
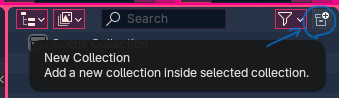
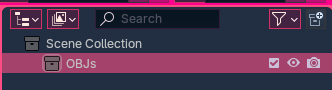
Import obj files
File > Import > Wavefron(.obj)
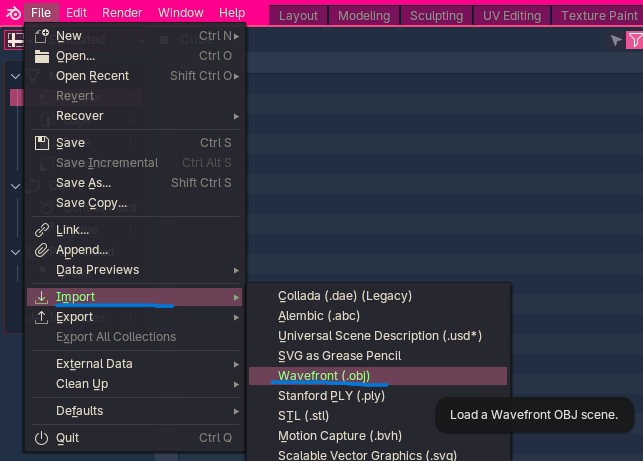
Select all obj files and load them into the collection you just created.
If you want to match the scale of the scene with AfterEffects Element3D, set the scale to 0.025x. The reason is that the scene scale in AE and Blender is 1:40.
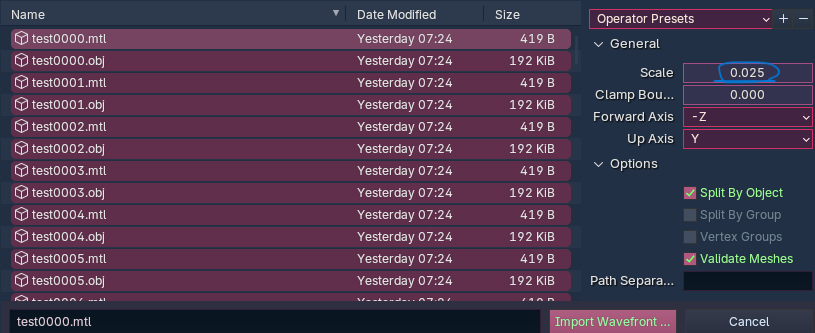
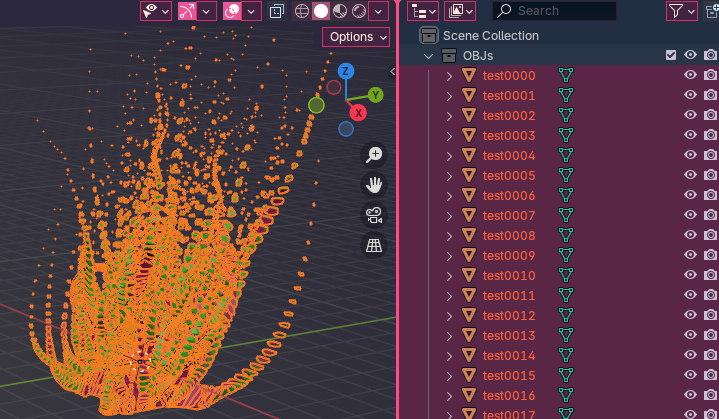
After loading the obj sequence, hide it.
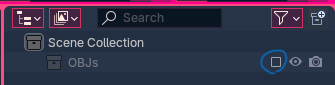
Applying Geometry Nodes
Create objects for Geometry Nodes. Any object can be used.
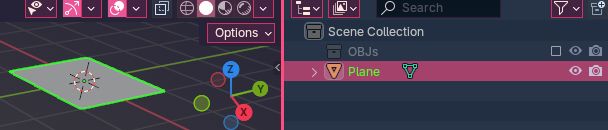
Apply Geometry Nodes.
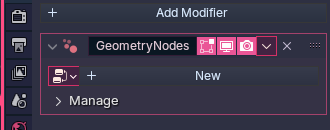
push new button
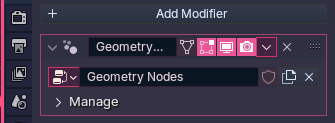
Applying the Script process
Preparation
Paste the script listed at the bottom of the article into a text tool and save it with the extension (.py).
Script tab

Open and run the saved python file
open the saved python file
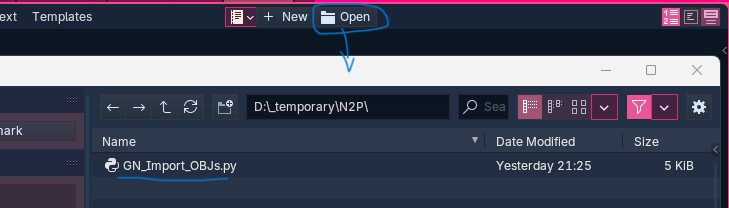
run the saved python file
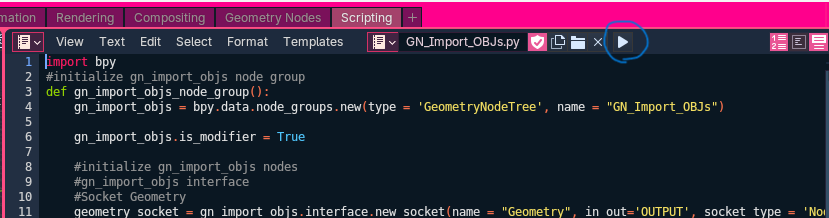
Choose Node Tree data
When the script is executed, Node Tree Data will be added to Geometry Nodes.
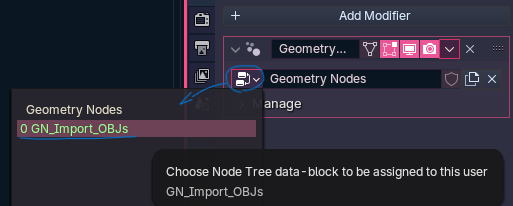
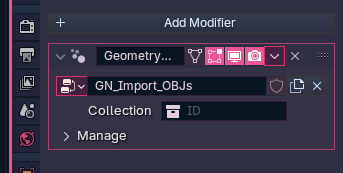
choose obj sequence collection
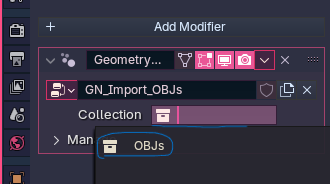
All Done!
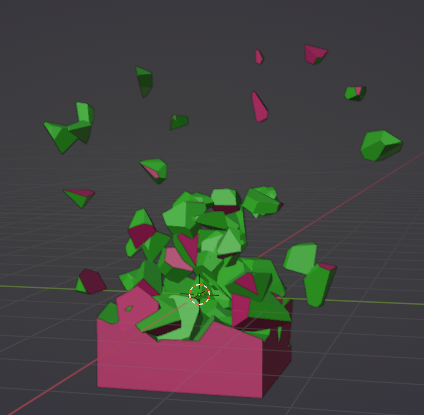
Script
Paste into text tool and save with extension (.py)
import bpy
#initialize gn_import_objs node group
def gn_import_objs_node_group():
gn_import_objs = bpy.data.node_groups.new(type = 'GeometryNodeTree', name = "GN_Import_OBJs")
gn_import_objs.is_modifier = True
#initialize gn_import_objs nodes
#gn_import_objs interface
#Socket Geometry
geometry_socket = gn_import_objs.interface.new_socket(name = "Geometry", in_out='OUTPUT', socket_type = 'NodeSocketGeometry')
geometry_socket.attribute_domain = 'POINT'
#Socket Geometry
geometry_socket_1 = gn_import_objs.interface.new_socket(name = "Geometry", in_out='INPUT', socket_type = 'NodeSocketGeometry')
geometry_socket_1.attribute_domain = 'POINT'
#Socket Collection
collection_socket = gn_import_objs.interface.new_socket(name = "Collection", in_out='INPUT', socket_type = 'NodeSocketCollection')
collection_socket.attribute_domain = 'POINT'
#node Group Input
group_input = gn_import_objs.nodes.new("NodeGroupInput")
group_input.name = "Group Input"
#node Group Output
group_output = gn_import_objs.nodes.new("NodeGroupOutput")
group_output.name = "Group Output"
group_output.is_active_output = True
#node Mesh Line
mesh_line = gn_import_objs.nodes.new("GeometryNodeMeshLine")
mesh_line.name = "Mesh Line"
mesh_line.count_mode = 'TOTAL'
mesh_line.mode = 'OFFSET'
#Count
mesh_line.inputs[0].default_value = 10
#Resolution
mesh_line.inputs[1].default_value = 1.0
#Start Location
mesh_line.inputs[2].default_value = (0.0, 0.0, 0.0)
#Offset
mesh_line.inputs[3].default_value = (0.0, 0.0, 0.0)
#node Mesh to Points
mesh_to_points = gn_import_objs.nodes.new("GeometryNodeMeshToPoints")
mesh_to_points.name = "Mesh to Points"
mesh_to_points.mode = 'VERTICES'
#Selection
mesh_to_points.inputs[1].default_value = True
#Position
mesh_to_points.inputs[2].default_value = (0.0, 0.0, 0.0)
#Radius
mesh_to_points.inputs[3].default_value = 0.05000000074505806
#node Instance on Points
instance_on_points = gn_import_objs.nodes.new("GeometryNodeInstanceOnPoints")
instance_on_points.name = "Instance on Points"
#Selection
instance_on_points.inputs[1].default_value = True
#Pick Instance
instance_on_points.inputs[3].default_value = True
#Rotation
instance_on_points.inputs[5].default_value = (0.0, 0.0, 0.0)
#Scale
instance_on_points.inputs[6].default_value = (1.0, 1.0, 1.0)
#node Collection Info
collection_info = gn_import_objs.nodes.new("GeometryNodeCollectionInfo")
collection_info.name = "Collection Info"
collection_info.transform_space = 'ORIGINAL'
#Separate Children
collection_info.inputs[1].default_value = True
#Reset Children
collection_info.inputs[2].default_value = False
#node Scene Time
scene_time = gn_import_objs.nodes.new("GeometryNodeInputSceneTime")
scene_time.name = "Scene Time"
#node Math
math = gn_import_objs.nodes.new("ShaderNodeMath")
math.name = "Math"
math.operation = 'SUBTRACT'
math.use_clamp = False
#Value_001
math.inputs[1].default_value = 1.0
#Value_002
math.inputs[2].default_value = 0.5
#Set locations
group_input.location = (-114.83021545410156, -291.7060852050781)
group_output.location = (529.0859375, -5.453728199005127)
mesh_line.location = (-119.99999237060547, -3.72674560546875)
mesh_to_points.location = (99.99999237060547, -7.5102386474609375)
instance_on_points.location = (321.3642578125, -4.681127071380615)
collection_info.location = (101.83811950683594, -206.2608642578125)
scene_time.location = (-107.96189880371094, -438.54559326171875)
math.location = (107.79608917236328, -390.6209716796875)
#Set dimensions
group_input.width, group_input.height = 140.0, 100.0
group_output.width, group_output.height = 140.0, 100.0
mesh_line.width, mesh_line.height = 140.0, 100.0
mesh_to_points.width, mesh_to_points.height = 140.0, 100.0
instance_on_points.width, instance_on_points.height = 140.0, 100.0
collection_info.width, collection_info.height = 140.0, 100.0
scene_time.width, scene_time.height = 140.0, 100.0
math.width, math.height = 140.0, 100.0
#initialize gn_import_objs links
#instance_on_points.Instances -> group_output.Geometry
gn_import_objs.links.new(instance_on_points.outputs[0], group_output.inputs[0])
#mesh_line.Mesh -> mesh_to_points.Mesh
gn_import_objs.links.new(mesh_line.outputs[0], mesh_to_points.inputs[0])
#mesh_to_points.Points -> instance_on_points.Points
gn_import_objs.links.new(mesh_to_points.outputs[0], instance_on_points.inputs[0])
#collection_info.Instances -> instance_on_points.Instance
gn_import_objs.links.new(collection_info.outputs[0], instance_on_points.inputs[2])
#group_input.Collection -> collection_info.Collection
gn_import_objs.links.new(group_input.outputs[1], collection_info.inputs[0])
#scene_time.Frame -> math.Value
gn_import_objs.links.new(scene_time.outputs[1], math.inputs[0])
#math.Value -> instance_on_points.Instance Index
gn_import_objs.links.new(math.outputs[0], instance_on_points.inputs[4])
return gn_import_objs
gn_import_objs = gn_import_objs_node_group()